Game Engine Toolset Development
Projects | | Links: Installer | Bonus Ch. 1 | Bonus Ch. 2 | Website | Amazon
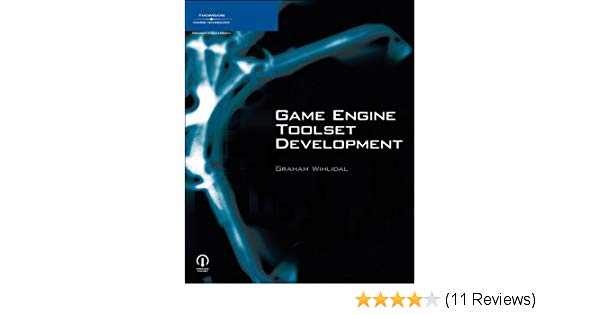
Game Engine Toolset Development
provides you with a core set of skills and a comprehensive insight that will aid you in the development of game engine utilities, significantly reducing the time period associated with the construction phase of a project. The book starts off with topics regarding development methodologies and best practices, and then proceeds into advanced topics like swap chain management and MVC object model automation with CodeDom.
An introductory working knowledge of C# and the .NET 2.0 framework is expected, allowing the content of each topic to be directed towards the subject and avoiding trivial and introductory explanations. Readers are not required to have any experience developing game engine tools. Terminology and design fundamentals specific to toolset development are clearly depicted and explained. The companion Web site provides downloads of all source code from the book, as well as several bonus chapters.
Details Permalink
- Paperback: 640 pages
- Released: 1st Edition (March 6, 2006)
- Publisher: Cengage Learning PTR;
- Language: English
- ISBN-10: 1592009638
- ISBN-13: 978-1592009633
Contents Permalink
Part 1. Toolset Design Fundamentals Permalink
- What is a Tool? What is a Toolset?
- Stakeholders: Internal Versus External
- Who Builds the Tools?
- How Large Are Tools Teams?
- Why Use C#? Why Use .NET?
- Overview of .NET
- Overview of C#
- Legacy Interoperability
- Benefits
- Examples of Commercial Toolsets
- Case Study: BioWare Corporation
- Case Study: Artificial Studios
- Everything Starts with a Plan
- Vision
- Stakeholders
- Reusability
- Architecture
- Requirements
- Design Standards
- Coding Standards
- Documentation
- Testing
- Defect Tracking
- Life Cycle
- Development Environment
- Staging Environment
- Production Environment
- Development Phases of a Tool
- Phase: Planning
- Phase: Analysis
- Phase: Design
- Phase: Implementation
- Measurement Metrics for Tool Quality
- Metric: Maintainability
- Metric: Traceability
- Metric: Performance
- Metric: Usability
- Metric: Testability
- Metric: Portability
- Metric: Reliability
- Metric: Efficiency
- Fundamentals of User Interface Design
- Principle of Consistency
- Principle of Transparency
- Principle of Feedback
- Principle of Refinement
- Principle of Exploration
- Principle of Modality
- Principle of Self-Evidence
- Principle of Moderation
- Principle of Customization
- Distributed Componential Architecture Design
- Architecture Overview
- Core Components
- Specific Tool Logic
- Console Entry Point
- Windows Entry Point
- Other Entry Points
- Architecture Example
- Alternate Architecture Structure
- Solutions to Bridge Domain Gaps
- Compositional Friction
- Cause: Domain Coverage
- Cause: Design Intentions
- Cause: Framework Gap
- Cause: Entity Overlap
- Cause: Legacy Components
- Cause: Source Code Access
- Relevant Design Patterns
- Unit Testing with NUnit
- Overview of Unit Testing
- Introducing NUnit
- Creating an NUnit Project
- Attribute Overview
- Expected Outcome Assertion
- A Simple Example
- Running Tests
- Debugging with Visual Studio
- Code Documentation with NDoc and XML
- Configuring the Project
- Supported XML Markup
- Commenting Example
- Generating the Documentation
- Microsoft Coding Conventions
- Styles of Capitalization
- Naming Classes
- Naming Interfaces
- Naming Namespaces
- Naming Attributes
- Naming Enumerations
- Naming Static Fields
- Naming Parameters
- Naming Methods
- Naming Properties
- Naming Events
- Abbreviations
- Enforcing Coding Policies with FxCop
- Installing FxCop
- Creating an FxCop Project
- Configuring Built-In Rules
- Analyzing Your Project
- Building Custom Rules
- Best Practices for Robust Exception Handling
- External Data Is Evil
- Creating Custom Exceptions
- Throwing Exceptions
- Structured Exception Handlers
- Logging Exception Information
- Mechanisms for Cleanup
- Unhandled and Thread Exception Events
Part 2. Techniques for Arbitrary Tools Permalink
- Compressing Data to Reduce Memory Footprint
- Types of Compression
- GZipStream Compression in .NET 2.0
- Implementation for Arbitrary Data
- Implementation for Serializable Objects
- Protecting Sensitive Data with Encryption
- Encryption Rudiments
- Selecting a Cipher
- ICryptoTransform Interface
- Generic Batch File Processing Framework
- Goals
- Proposed Solution
- Implementation
- Ensuring a Single Instance of an Application
- Early Solutions
- Journey to the Dark Side
- The Solution
- Implementing a Checksum to Protect Data Integrity
- Implementation
- Usage
- Alternative
- Using the Property Grid Control with Late Binding
- Designing a Bindable Class
- Ordering Properties
- Using the PropertyGrid
- Adding Printing Support for Arbitrary Data
- Printing Regular Text
- Supporting Printer Selection
- Supporting Page Setup
- Supporting Print Preview
- Flexible Command Line Tokenizer
- Formatting Styles
- Implementation
- Sample Usage
- Layering WinForms on Console Applications
- Implementation
- Sample Usage
- Overview of Database Access with ADO.NET
- Advantages of ADO.NET
- ADO.NET Object Model
- Working with a DataReader
- Working with a DataAdapter
- Working with XML
- Potion Database Editor
Part 3. Techniques for Graphical Tools Permalink
- Using Direct3D Swap Chains with MDI Applications
- What is a Swap Chain?
- Thoughts for SDI and MDI Applicability
- Common Pitfalls
- The Proposed Solution
- Constructing an Aesthetic Texture Browser Control
- Swappable Loader Interface
- Windows GDI+ Loader
- Managed Direct3D Loader
- Storing Texture Information
- Building the Thumbnail Control
- Handling Custom User Events
- Building the Viewer Control
- Using the Control
- Loading Textures from a Directory
- Loading Textures from a MemoryStream
- Loading Textures from a Bitmap
- Texture Browser Demo
- Converting from Screen Space to World Space
- Transforming Screen Coordinates
- Computing the Picking Ray
- Bounding Sphere Intersection Tests
- Improving Intersection Accuracy
- Using Built-In D3DX Functionality
- Asynchronous Input Device Polling
- Asynchronous Mouse Polling
- Asynchronous Keyboard Polling
- Sample Usage
Part 4. Techniques for Network Tools Permalink
- Downloading Network Files Asynchronously
- HttpWebRequest and HttpWebResponse
- The Request Object
- Maintaining Data State
- The Core System
- Sample Usage
Part 5. Techniques for Legacy Interoperability Permalink
- Exchanging Data between Applications
- What Microsoft.NET Provides
- Web Services
- Remoting
- Clipboard
- TCP\IP Loopback Communication
- What Microsoft.NET Should Provide
- Named Pipes
- WM_COPYDATA
- Building a Wrapper Around WM_COPYDATA
- Communicating from Unmanaged Applications
- Communicating from Managed Applications
- Conclusion
- What Microsoft.NET Provides
- Interacting with the Clipboard
- The Clipboard Class and IDataObject
- Storing Built-In Types
- Storing Custom Data Formats
- Querying Available Data Formats
- Complete Solution
- Conclusion
- Using .NET Assemblies as COM Objects
- COM Callable Wrappers (CCW)
- Applying Interop Attributes
- Registering with COM
- Accessing from Unmanaged Code
- Deployment Considerations
- Conclusion
- Managing Items in the Recent Documents List
- Implementation
- Example Usage
- Conclusion
Part 6. Techniques to Improve Performance Permalink
- Playing Nice with the Garbage Collector
- Overview of the Garbage Collector
- Collecting the Garbage
- Allocation Profile
- CLR Profiler and GC Monitoring
- Performance Counters
- Profiling API and the CLR Profiler
- Finalization and the Dispose Pattern
- Finalization
- The Dispose Pattern
- Weak Referencing
- Explicit Control
- Conclusion
- Using Unsafe Code and Pointers
- Rudiments of Pointer Notation
- Using an Unsafe Context
- Pinning Memory with the Fixed Statement
- Disabling Arithmetic Overflow Checking
- Allocating High Performance Memory
- Getting Size of Data Types
- Example: Array Iteration and Value Assignment
- Example: Data Block Copying
- Example: Win32 API Access
- Conclusion
- Investigating Managed Code Performance
- Investigating Performance
- Avoid Manual Optimization
- String Comparison
- String Formatting
- String Reversal
- Compiling Regular Expressions
- Use the Most Specific Type
- Avoid Boxing and Unboxing
- Use Value Types Sensibly
- The Myth About Foreach Loops
- Use Asynchronous Calls
- Efficient IO Buffer Sizes
- Minimize the Working Set
- Perform Chunky Calls
- Minimize Exception Throwing
- Thoughts About NGen
- Conclusion
- Responsive UI during Intensive Processing
- Implementing the Worker Logic
- Reporting Operation Progress
- Supporting User Cancellation
- Executing the Worker Thread
- Conclusion
Part 7. Techniques to Enhance Usability Permalink
- Designing an Extensible Plugin-Based Architecture
- Designing a Common Interface
- Embedding Plugin Metadata Information
- Building a Proxy Wrapper
- Loading Plugins Through the Proxy
- Reloading Plugins During Runtime
- Runtime Compilation of Plugins
- Enforcing a Security Policy
- Conclusion
- Persisting Application Settings to Isolated Storage
- Concept of Isolated Storage
- Accessing Isolated Storage
- Levels of Isolation
- Management and Debugging
- Conclusion
- Desiging a Reusable and Versatile Loading Screen
- Splash Dialog
- Go for the Gusto
- Concept of Loading Jobs
- Responsive Processing
- Simple Example
- Conclusion
- Writing Context Menu Shell Extensions
- Unmanaged Interfaces
- Reusable Framework
- Sample Usage—Standalone
- Sample Usage—Integrated
- Component Registration
- Debugging Techniques
- Conclusion
Part 8. Techniques to Increase Productivity Permalink
- Automating Workflow using Job Scheduling
- Benefits
- Solution Goals
- Implementation
- Conclusion
- MVC Object Model Automation with CodeDom
- Advantages of an Automatable Object Model
- Comparison with Model-View-Controller Pattern
- A Simple Object Model Architecture
- Plugin-Based Architectures
- Controlling an Object Model with Scripts
- Implementing a C# Command Window
- Simple Automation and MVC Example
- Conclusion
Part 9. Techniques for Deployment and Support Permalink
- Deployment and Versioning with ClickOnce
- ClickOnce and MSI Comparison
- Creating the Application
- Publishing the Application
- Launching the Application
- Deployment Configuration
- Pushing Application Updates
- Programmatically Handling Updates
- Conclusion
- Testing for the Availability of the .NET Framework
- The Solution
- Example Usage
- Conclusion
- Building and Customizing an MSI Installer
- Creating a Setup Project
- Project Configuration
- Deployment Configuration
- Custom Installer Actions
- Deploying the Installer
- Conclusion
- Determining Binary File Differences
- What is Levenshtein Distance?
- Generating a Difference List
- Transforming Data Using a Difference List
- Thoughts for Usability and Deployment
- Conclusion
Part 10. Bonus Web Site Chapters Permalink
- Distributed Computing using .NET Remoting
- Investigating Scalability
- Fault Tolerance
- Managing Security
- Middleware Considerations
- Sample Framework
- Conclusion
- Building a Managed Wrapper with C++/CLI
- Introduction to Managed C++ (C++/CLI)
- Overview of Extended Syntax
- Reference Handles
- Keyword: abstract
- Keyword: delegate
- Keyword: event
- Keyword: interface
- Keyword: property
- Keyword: sealed
- Keyword: __identifier
- Keyword: pin_ptr
- Keyword: safe_cast
- Keyword: typeid
- Common Language Runtime Compilation (/clr)
- Referencing Assemblies and Classes
- Mixing Managed and Unmanaged Code
- Overview of Extended Syntax
- Example Unmanaged 3D Engine
- Creating a Managed Wrapper for SimpleEngine
- Consuming the Managed Wrapper
- Conclusion
- Introduction to Managed C++ (C++/CLI)